Dymaxion world map with proportional circles and automatic legend in D3.js v5
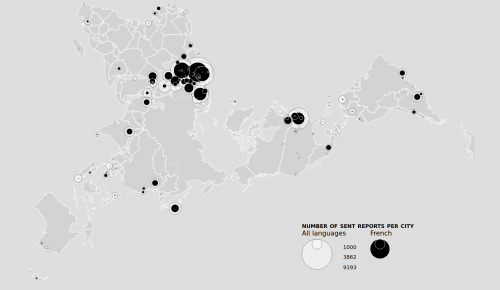
virtualenvwrapper is a set of extensions to Ian Bicking’s virtualenv tool. The extensions include wrappers for creating and deleting virtual environments and otherwise managing your development workflow, making it easier to work on more than one project at a time without introducing conflicts in their dependencies.
Features
Organizes all of your virtual environments in one place.
Wrappers for managing your virtual environments (create, delete, copy).
Use a single command to switch between environments.
Tab completion for commands that take a virtual environment as argument.
User-configurable hooks for all operations (see Per-User Customization).
Plugin system for creating more sharable extensions (see Extending Virtualenvwrapper).
This guide discusses how to install packages using pip and a virtual environment manager: either venv for Python 3 or virtualenv for Python 2. These are the lowest-level tools for managing Python packages and are recommended if higher-level tools do not suit your needs.
Well, a virtual environment is just a directory with three important components:
A site-packages/ folder where third party libraries are installed.
Symlinks to Python executables installed on your system.
Scripts that ensure executed Python code uses the Python interpreter and site packages installed inside the given virtual environment.
(venv) % pip freeze numpy==1.15.3
And write the output to a file, which we’ll call requirements.txt.
(venv) % pip freeze > requirements.txt
Duplicating Environments
Sara% cd test-project/ Sara% python3 -m venv venv/ (venv) Sara% pip install -r requirements.txtCollecting numpy==1.15.3 (from -r i (line 1)) Installing collected packages: numpy Successfully installed numpy-1.15.3
…python -m pip executes pip using the Python interpreter you specified as python. So /usr/bin/python3.7 -m pip means you are executing pip for your interpreter located at /usr/bin/python3.7.
But when you use python -m pip with python being the specific interpreter you want to use, all of the above ambiguity is gone. If I say python3.8 -m pip then I know pip will be using and installing for my Python 3.8 interpreter (same goes for if I had said python3.7).
While we’re on the subject of how to avoid messing up your Python installation, I want to make the point that you should never install stuff into your global Python interpreter when you. develop locally (containers are a different matter)! If it’s your system install of Python then you may actually break your system if you install an incompatible version of a library that your OS relies on.
Building a truly international application is not just about translating strings. Other issues to consider are date and time formats, currency symbols and pluralization. Programmers often underestimate the complexity of localization and get stuck with homemade code that is a pain to maintain. So, let’s talk about PHP Arrays, gettext, frameworks, and Intl.
First I take the whole CSV file and split it into an array of lines. Then, I take the first line, which should be the headers, and split that by a comma into an array. Then, I loop through all the lines (skipping the first one) and inside, I loop through the headers and assign the values from each line to the proper object parameters. At this point, you probably want to just return the JavaScript object, but you can also JSON.stringify the result and return the JSON object.
5.x implementation of a choropleth map, using Promises.
A pretty specific title, huh? The versioning is key in this map-making how-to. D3.js version 5 has gotten serious with the Promise class which resulted in some subtle syntax changes that proven big enough to cause confusion among the D3.js old dogs and the newcomers. This post guides you through creating a simple map in this specific version of the library. If you’d rather dive deeper into the art of making maps in D3 try the classic guides produced by Mike Bostock.
A specification for adding human and machine readable meaning to commit messages.
The Conventional Commits specification is a lightweight convention on top of commit messages. It provides an easy set of rules for creating an explicit commit history; which makes it easier to write automated tools on top of. This convention dovetails with SemVer, by describing the features, fixes, and breaking changes made in commit messages.
The Customizer Export/Import plugin allows you to export or import your WordPress customizer settings from directly within the customizer interface! If your theme makes use of the WordPress customizer for its settings, this plugin is for you!
To create a «polls» app in the «apps» sub-directory, do the following first to make the directory (assuming you are at the root of your django project):
mkdir apps/polls
Next, run startapp to create «polls» in under the «app» project directory.
startapp polls apps/polls
Install the app;
INSTALLED_APPS = [
‘apps.polls’
]
migrate:
makemigrations polls
migrate
optional:
main urls.py
urlpatterns = [
path(‘polls/’,include(‘apps.polls.urls’),
]
welcome to the year 2030.
there are 1.2 billion more people on the planet.
70% of us are living in cities now.
in order to house 1.2 billion more people,
all of us are sharing more household goods and services than ever before.
we refer to this sharing as co-living.
and many more of us are living this way now.
but it’s not a new thing.
communal living has always been a solution to common problems.
like rapid urbanisation, loneliness, and high living costs.
but what does co-living look like in the year 2030?
who is it for?
how has it changed our society?
what are we sharing?
with many more of us now co-living,
there is no one configuration.
discover what type of co-living would be uniquely suited for you.
reserve your spot for ONE SHARED HOUSE 2030.
REM to PX Converter is a free online tool you can use to easily convert rem (root em) to px (pixel). Keep in mind that 1rem is equal to the root font-size, in other words, the font-size on element.
This tutorial explains how to create new files and directories in the WordPress /uploads/ folder.
Distributor is a WordPress plugin that makes it easy to syndicate and reuse content across your websites — whether in a single multisite or across the web.
const arrayToObject = (array) => array.reduce((obj, item) => { obj[item.id] = item return obj }, {})const peopleObject = arrayToObject(peopleArray) console.log(peopleObject[idToSelect])
We inspire women to fall in love with programming.
Django Girls organize free Python and Django workshops, create open sourced online tutorials and curate amazing first experiences with technology.
Django Girls is a non-profit organization and a community that empowers and helps women to organize free, one-day programming workshops by providing tools, resources and support. We are a volunteer run organization with hundreds of people contributing to bring more amazing women into the world of technology. We are making technology more approachable by creating resources designed with empathy.
During each of our events, 30-60 women build their first web application using HTML, CSS, Python and Django.
https://www.youtube.com/watch?v=8YP_nOCO-4Q
Building cross-platform desktop applications comes with a unique set of challenges that can stand in your way when you are trying to transform your ideas into software. Web apps avoid some of these hurdles, but they have limitations that make them impractical for building native desktop applications. Electron lets you harness the best parts of these technologies to build beautiful, cross-platform desktop applications using HTML, JavaScript, and CSS.
There are only two steps required: updating our articles/models.py file and articles/urls.py.
In our model, we can add Django’s built-in SlugField. But we must also–and this is the part that typically trips people up–update get_absolute_url as well. That’s where we pass in the value used in our URL.
A javascript component to create terrific cartography ! GoGoCartoJs is the autonomous frontend part of the GoGoCarto Project
The loading attribute allows a browser to defer loading offscreen images and iframes until users scroll near them. loading supports three values:
lazy: is a good candidate for lazy loading.
eager: is not a good candidate for lazy loading. Load right away.
auto: browser will determine whether or not to lazily load.
Not specifying the attribute at all will have the same impact as setting loading=auto.
Native lazy loading of images and iframes is super cool. Nothing could be more straightforward than the markup below:
<img src="myimage.jpg" loading="lazy" alt="..." /> <iframe src="content.html" loading="lazy"></iframe>
As you can see, no JavaScript, no dynamic swapping of the src attribute’s value, just plain old HTML.
The loading attribute gives us the option to delay off-screen images and iframes until users scroll to their location on the page. loading can take any of these three values:
lazy: works great for lazy loading
eager: instructs the browser to load the specified content right away
auto: leaves the option to lazy load or not to lazy load up to the browser.
After reading this article, you will get:
How to write SCSS/SASS using NPM for your Django project
How to deploy Django project which uses NPM as front-end solution
After reading this article, you will get:
The difference between CSS, SCSS, SASS
How to make Bootstrap SCSS work in your Django project.
How to deploy SCSS in Django project.
DevOps is the combination of application development and operations, which minimizes or eliminates the disconnect between software developers who build applications and systems administrators who keep infrastructure running.
The mod_wsgi package implements a simple to use Apache module which can host any Python web application which supports the Python WSGI specification.
COVID-19 (2019 novel Coronavirus) is a current epidemic as of today. Developers around the world are building applications for the public to get up-to-date and accurate information as quickly as possible.
If you are a developer, you may also be able to contribute to some of these projects.
Using intersection observer
If you’ve written lazy loading code before, you may have accomplished your task by using event handlers such as scroll or resize. While this approach is the most compatible across browsers, modern browsers offer a more performant and efficient way to do the work of checking element visibility via the intersection observer API.
First of all, don’t use var. There are a few differences between var, let and const and the most important one to me is that let and const remove the error-prone behavior with variable hoisting.
Unlike other search engines, DuckDuckGo doesn’t offer any kind of webmaster tools.
Duckduckgo also not offers URL submission.
So, Is there any way to submit your site to DuckDuckGo. Yes, they don’t have any kind of a crawler that is going to crawl your website. To index your site, you have to rely on the search results from other search engines and come up with a way to index your site.
There is no need to submit your site to duckduckgo also there is no way to submit.
As duckduckgo automatically index your site.
So, It might take some time to show your site on duckduckgo search Engine.
I have made a Youtube Tutorial on Google, Bing and Yandex Webmaster Submission. Have a look at that.
It only does PNG, but Firefox has a way to capture the whole page built in: Shift-F2 brings up a command prompt, which includes a screenshot command. For instance, screenshot –clipboard –fullpage as I was writing this answer produced http://imgur.com/tnplKPE.
If you want something designed to be automated, phantomjs has page.render() which takes a filename and an optional options object, with format and quality entries; the example given is
page.render('google_home.jpeg', {format: 'jpeg', quality: '100'});
Download an image from the specified URL and attach it to a post.
media_sideload_image($file, $post_id, $desc, $return);
TL;DR:
itty.bitty takes html (or other data), compresses it into a URL fragment, and provides a link that can be shared. When it is opened, it inflates that data on the receiver’s side.
…self-contained microsites that exist solely as URLs
Simply the Best PHP & Symfony Tutorials
And the official way to learn Symfony
Sample-Videos.com is a 100% FREE service that allows programmers, testers, designers, developers to download sample videos for demo/test use. No matter what video format they use (MP4, FLV, MKV, 3GP); they will be able to test videos on any Smartphone without any hustle. This is a one stop destination for all sample video testing needs. Just search below for the relevant video formats in specific sizes, download them, and start testing.
A mobile screen resolution can be a big challenge when it comes to watching videos online or offline. By testing out videos one can be rest assured regarding the video playback in an app, without annoying the end users. Choose from four different video extensions i.e. 3GP, MKV, FLV, and MP4. The best thing is all of these are free and very easy to download. We provide you with a specific and perfect resolution in all sizes possible with just one simple click. Check out the one which suits your requirements. All you need to do is hit the DOWNLOAD button, and get started.
The pushState() method let’s you update the URL and create a new item in the browser history without reloading the page. Because the history is updated, this new URL can be changed with the browser’s forward and backward buttons as well.
Here’s how it works.
history.pushState(state, pageTitle, url);
Syntastic is a syntax checking plugin for Vim created by Martin Grenfell. It runs files through external syntax checkers and displays any resulting errors to the user. This can be done on demand, or automatically as files are saved. If syntax errors are detected, the user is notified and is happy because they didn’t have to compile their code or execute their script to find them.
Images with only visual value should have an empty alt attribute set on them. Omitting the alt attribute makes most screen readers read out the entire image URL and providing an alt attribute when the image is for visual purposes only is just as useless.